Dev C++ For Loop Counter
The most common form of loop in C++ is the for loop. The for loop is preferred over the more basic while loop because it’s generally easier to read (there’s really no other advantage).
For Loop Counter
C 'for' loops aren't quite as easy to apply that optimization to as Pascal 'for' loops are because the bounds of Pascal's loops are always fully calculated before the loop runs, whereas C loops sometimes depend on the stopping condition and the loop contents. C compilers need to do some amount of static analysis to determine whether any.
The for loop has the following format:
- Unlike for and while loops, which test the loop condition at the top of the loop, the do.while loop checks its condition at the bottom of the loop. A do.while loop is similar to a while loop, except that a do.while loop is guaranteed to execute at least one time. The syntax of a do.while loop in C is −.
- C for Loop Loops are used in programming to repeat a specific block of code. In this tutorial, you will learn to create a for loop in C programming (with examples). Loops are used in programming to repeat a specific block until some end condition is met. There are three type of loops in C programming.
- May 30, 2017 C example introducing while loops with very basic counter controlled loop. C example introducing while loops with very basic counter controlled loop. Skip navigation.
The for loop is equivalent to the following while loop:
Stream PUMPER - The Ultimate 3 in 1 Tool VST, VST3, AU OUT NOW!! Production® from desktop or your mobile device. Pumper is available via PluginBoutique (€28 / $29) website for both Windows and MAC hosted apps in VST, VST3, AU (32bit & 64bit) plugin formats. BONUS: FREE Exclusive W.A Production Sample Pack. For more details about this plugin please check the link below. Pumper vst free download free. Production Pumper Stereo Image v1.0.1 Free Download. Click on below button to start W. Production Pumper Stereo Image v1.0.1 Free Download. This is complete offline installer and standalone setup for W. Production Pumper Stereo Image v1.0.1. This would be compatible with both 32 bit and 64 bit windows. Production Pumper Saturator v1.0.1 Free Download. Click on below button to start W. Production Pumper Saturator v1.0.1 Free Download. This is complete offline installer and standalone setup for W. Production Pumper Saturator v1.0.1. This would be compatible with both 32 bit and 64 bit windows. Nov 27, 2014 Free VST – One Knob Pumper. Posted on Nov 27, 2014. Free New Plugin – OneKnob Pumper – Waves. OneKnob Pumper. Instant ducking effect. Get it here —- DOWNLOAD. Designed for achieving an instant ducking effect, OneKnob Pumper simulates sidechain compression, saving you the need to route the kick track to the sidechain.
Execution of the for loop begins with the initialization clause, which got its name because it’s normally where counting variables are initialized. The initialization clause is executed only once, when the for loop is first encountered.
Execution continues with the conditional clause. This clause works just like the while loop: As long as the conditional clause is true, the for loop continues to execute.
After the code in the body of the loop finishes executing, control passes to the increment clause before returning to check the conditional clause — thereby repeating the process. The increment clause normally houses the autoincrement or autodecrement statements used to update the counting variables.
The for loop is best understood by example. The following ForDemo1 program is nothing more than the WhileDemo converted to use the for loop construct:
The program reads a value from the keyboard into the variable nloopCount. The for starts out comparing nloopCount to 0. Control passes into the for loop if nloopCount is greater than 0. Once inside the for loop, the program decrements nloopCount and displays the result. That done, the program returns to the for loop control.
Control skips to the next line after the for loop as soon as nloopCount has been decremented to 0.
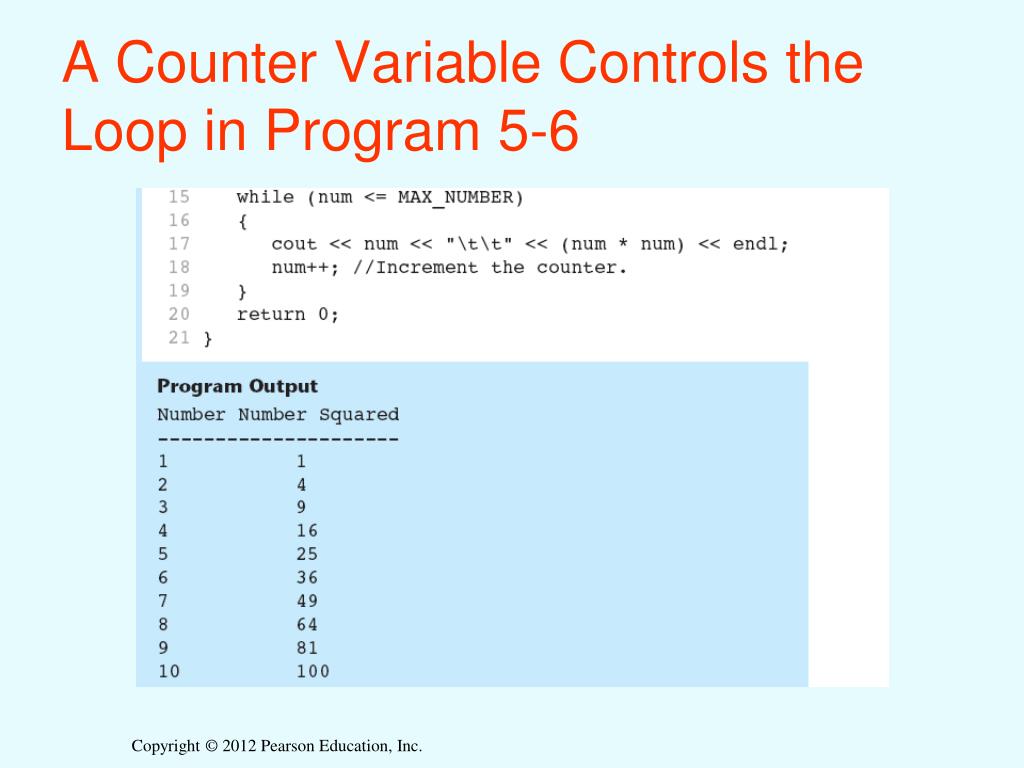
All three sections of a for loop may be empty. An empty initialization or increment section does nothing. An empty comparison section is treated like a comparison that returns true.

This for loop has two small problems. First, it’s destructive, in the sense that it changes the value of nloopCount, “destroying” the original value. Second, this for loop counts backward from large values down to smaller values. These two problems are addressed by adding a dedicated counting variable to the for loop. Here’s what it looks like:
This modified version of ForDemo loops the same as it did before. Instead of modifying the value of nLoopCount, however, this ForDemo2 version uses a new counter variable.
This for loop declares a counter variable i and initializes it to 0. It then compares this counter variable to nLoopCount. If i is less than nLoopCount, control passes to the output statement within the body of the for loop. Once the body has completed executing, control passes to the increment clause where i is incremented and compared to nLoopCount again, and so it goes.
The following shows example output from the program:
When declared within the initialization portion of the for loop, the index variable is known only within the for loop itself. Nerdy C++ programmers say that the scope of the variable is limited to the for loop. In the ForDemo2 example just given, the variable i is not accessible from the return statement because that statement is not within the loop.
Language | ||||
Standard Library Headers | ||||
Freestanding and hosted implementations | ||||
Named requirements | ||||
Language support library | ||||
Concepts library(C++20) | ||||
Diagnostics library | ||||
Utilities library | ||||
Strings library | ||||
Containers library | ||||
Iterators library | ||||
Ranges library(C++20) | ||||
Algorithms library | ||||
Numerics library | ||||
Input/output library | ||||
Localizations library | ||||
Regular expressions library(C++11) | ||||
Atomic operations library(C++11) | ||||
Thread support library(C++11) | ||||
Filesystem library(C++17) | ||||
Technical Specifications |
Labels | ||||
label : statement | ||||
Expression statements | ||||
expression ; | ||||
Compound statements | ||||
{ statement.. } | ||||
Selection statements | ||||
if | ||||
switch | ||||
Iteration statements | ||||
while | ||||
do-while | ||||
for | ||||
range for(C++11) | ||||
Jump statements | ||||
break | ||||
continue | ||||
return | ||||
goto | ||||
Declaration statements | ||||
declaration ; | ||||
Try blocks | ||||
try compound-statementhandler-sequence | ||||
Transactional memory | ||||
synchronized , atomic_commit , etc(TM TS) |
Executes init-statement once, then executes statement and iteration_expression repeatedly, until the value of condition becomes false. The test takes place before each iteration.
[edit]Syntax
formal syntax: |
attr(optional)for ( init-statementcondition(optional); iteration_expression(optional)) statement |
informal syntax: |
attr(optional)for ( declaration-or-expression(optional); declaration-or-expression(optional); expression(optional)) statement |
attr(C++11) | - | any number of attributes |
init-statement | - | either
|
condition | - | either
|
iteration_expression | - | any expression, which is executed after every iteration of the loop and before re-evaluating condition. Typically, this is the expression that increments the loop counter |
statement | - | any statement, typically a compound statement, which is the body of the loop |
[edit]Explanation
The above syntax produces code equivalent to:
{
|
Except that
If the execution of the loop needs to be terminated at some point, break statement can be used as terminating statement.
If the execution of the loop needs to be continued at the end of the loop body, continue statement can be used as shortcut.
As is the case with while loop, if statement is a single statement (not a compound statement), the scope of variables declared in it is limited to the loop body as if it was a compound statement.
[edit]Keywords
[edit]Notes
C# For Loop Step Value
As part of the C++ forward progress guarantee, the behavior is undefined if a loop that has no observable behavior (does not make calls to I/O functions, access volatile objects, or perform atomic or synchronization operations) does not terminate. Compilers are permitted to remove such loops.
While in C++, the scope of the init-statement and the scope of statement are one and the same, in C the scope of statement is nested within the scope of init-statement:
[edit]Example
C For Loop
Output:
[edit]See also
range-for loop | executes loop over range (since C++11)[edit] |
C documentation for for |